Interacting with smart contracts using Golang
Generating a Go package with abigen tool to interact with smart contracts and perform contract operations
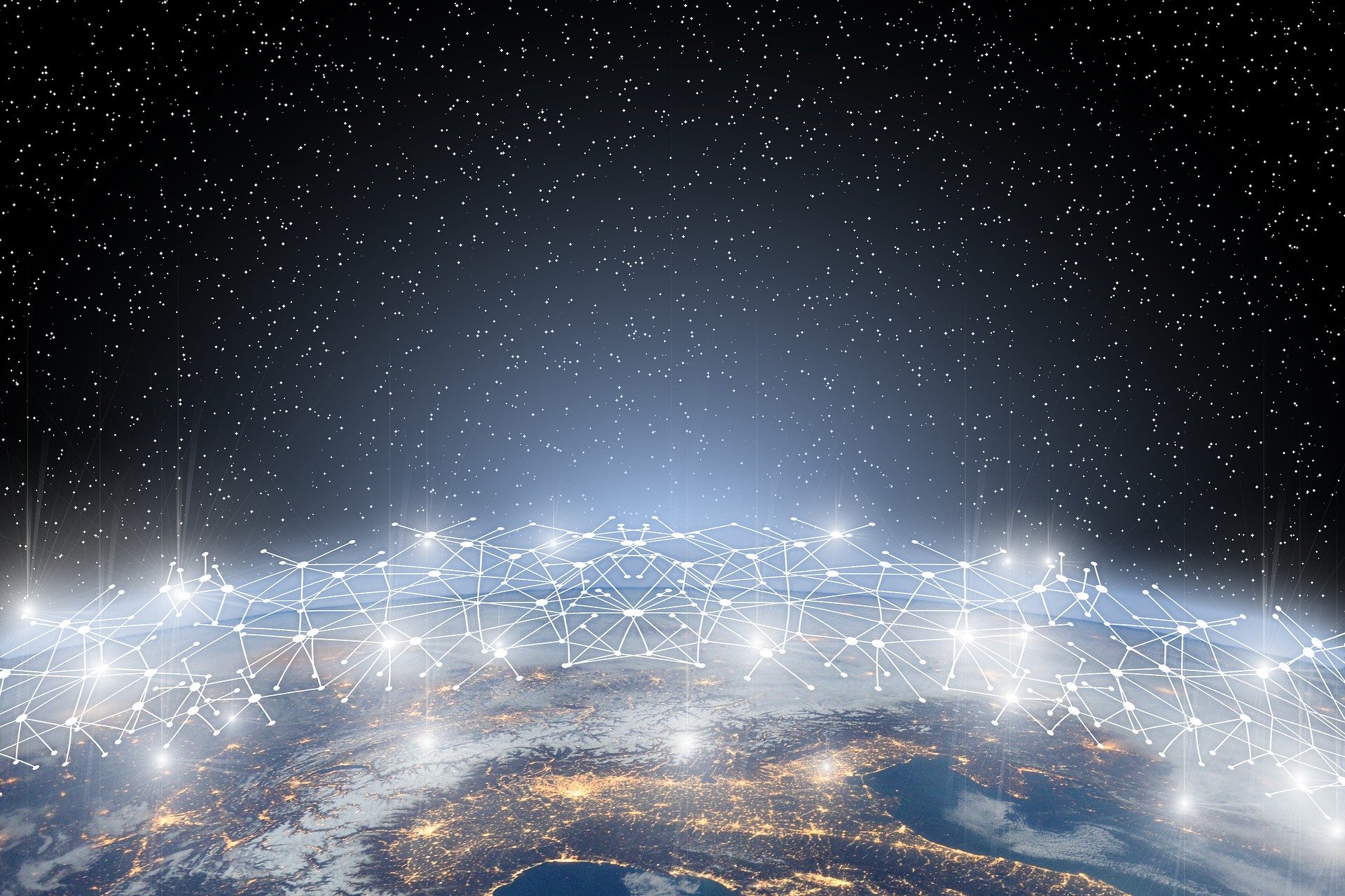
Previously, I was calling Smart Contracts through PHP, which raised two issues.
One was the slowness, and the other was the lack of maintenance of the package.
Fortunately, Golang provides an official package that is both fast and solves these two problems.
This article explains how to convert existing Smart Contract ABIs into a Go package for interacting with contracts.
Preparation
To set up the necessary packages on Mac, you need to install the following dependencies through Homebrew beforehand.
brew update
brew tap ethereum/ethereum
brew install ethereum
brew install solidity
brew install protobuf
Otherwise, you may encounter issues in the subsequent steps.
Please install solc
Please install protoc
Install go-ethereum
Next, you need to install the conversion tool we will be using.
The official package comes with the abigen
command, which allows you to convert the ABI into a Go package.
git clone https://github.com/ethereum/go-ethereum.git
cd go-ethereum
make devtools
Generate Go library file
Once the installation is complete, you will need the smart contract ABI to perform the conversion.
However, the topic of what a smart contract ABI is falls outside the scope of this article.
If you require a more in-depth explanation of smart contract operations in the future, it can be covered separately.
Once you have obtained the abi.json
file for the smart contract, you can proceed with the following command execution:
abigen --abi="./erc721.abi.json" --type="erc721" --pkg=erc721 --out="erc721.go"
flags | description | usage |
---|---|---|
–abi | file path for smart contract abi.json | ./erc721.abi.json |
–type | type name in struct | erc721 |
–pkg | go package name in output file | erc721 |
–out | output file name | erc721.go |
As a result, you will have a file named erc721.go
, and upon opening it, you will find that the package name is erc721
.
By comparing the functions inside the file, you will notice that they correspond to the functions in the abi.
Now, you can interact with the contract by importing and referencing the erc721
package.
Using the package in Golang.
To interact with the generated erc721
package in Go, you need to first import the package in your code.
Since blockchain operations are essential, you should also import go-ethereum
. Here’s an example:
import (
erc721 "project/package-erc721/path"
"github.com/ethereum/go-ethereum/common"
"github.com/ethereum/go-ethereum/ethclient"
)
Then interacting with smart contracts using go-ethereum.
The following example demonstrates the interaction with the TotalSupply
function in a smart contract.
Passing a smart contract’s contract address string: hexAddress
.
You can obtain the total supply issued by the contract, and this example focuses on an ERC721 NFT contract.
infuraUrl := "https://mainnet.infura.io/v3/38ad7d4b...97aa1d5c583"
client, err := ethclient.Dial(infuraUrl)
if err != nil {
return nil, err
}
defer client.Close()
address := common.HexToAddress(hexAddress)
instance, err := erc721.NewErc721(address, client)
if err != nil {
return nil, err
}
totalSupply, err := instance.TotalSupply(nil)
if err != nil {
return nil, err
}
return totalSupply, nil
This post illustrates how to use abigen
to generate a corresponding Go package for a Smart Contract.
So far, the functions called, including Owner
, OwnerOf
, and TotalSupply
, have successfully retrieved data from the blockchain.
There are other applications to explore in the future as opportunities arise for further operations.