Different between pointer and value for struct type in golang
The different between pointer and value for struct type in golang with examples
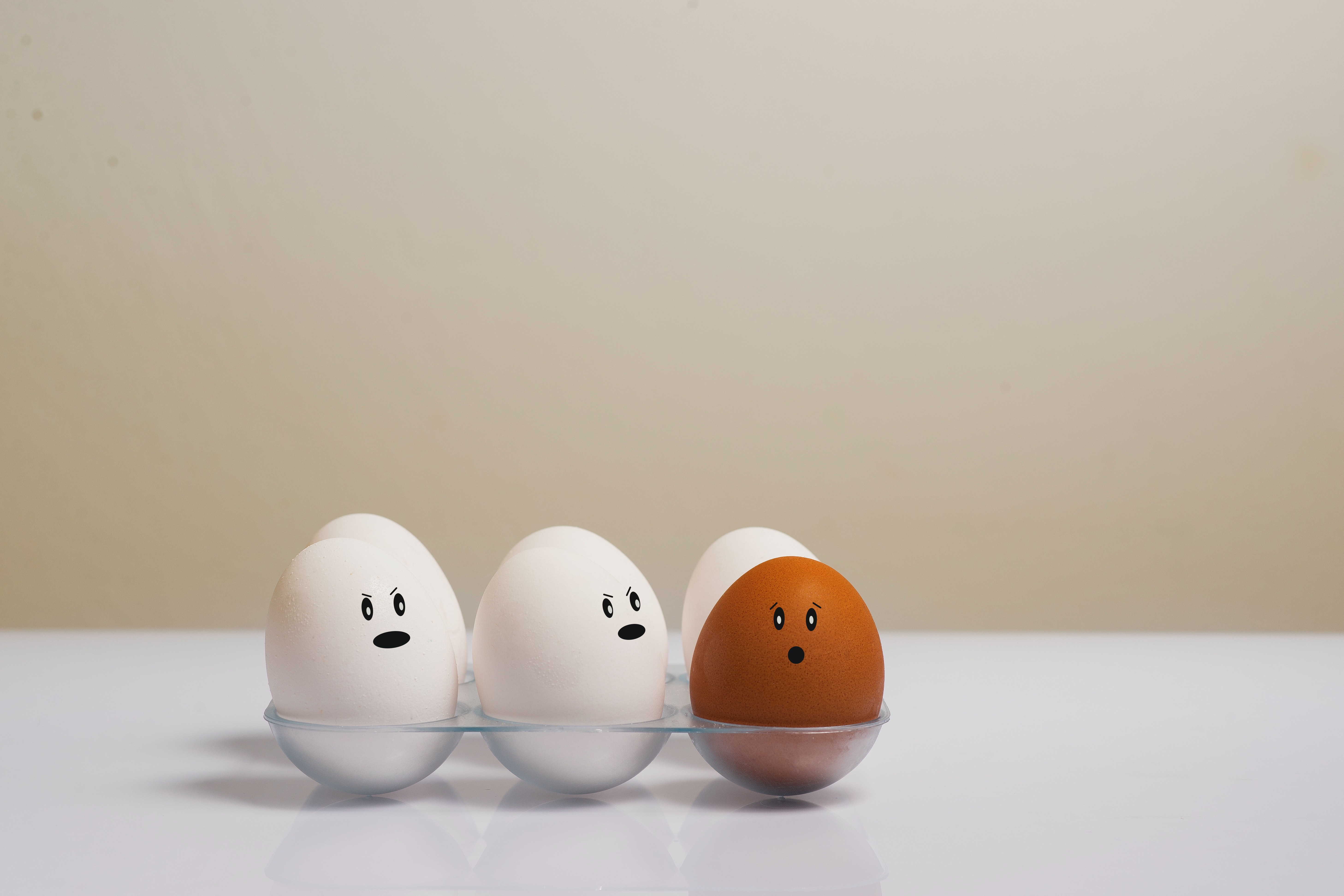
I always had a question “Should I use value for type in struct? Or using pointer?”
After reading many different projects on the web, it seems that they all have different practices.
Until I recently encountered a problem that gave me some ideas.
In our projects, we often start by defining the Request incoming Struct
Then use json.Unmarshal
to convert the incoming json string into Struct for subsequent operations
Previously, the default value for fields was false, so we pass by value.
In this case, the default value is true and the problem happened.
Take the first look at the code and execution results
package main
import (
"encoding/json"
"fmt"
)
type John struct {
Name string `json:"name"`
CanRun bool `json:"canRun"`
CanFly bool `json:"canFly"`
}
type Doe struct {
Name string `json:"name"`
CanRun *bool `json:"canRun"`
CanFly *bool `json:"canFly"`
}
func main() {
var param = []byte(`{"Name":"John Doe", "canRun":true}`)
defult := true
var john = new(John)
json.Unmarshal(param, &john)
jsondata, _ := json.Marshal(john)
fmt.Println(string(jsondata))
var doe = new(Doe)
json.Unmarshal(param, &doe)
doejsondata, _ := json.Marshal(doe)
fmt.Println(string(doejsondata))
if doe.CanFly == nil {
doe.CanFly = &defult
}
doejsondata, _ = json.Marshal(doe)
fmt.Println(string(doejsondata))
}
In the example, there are two Structs.
John, whose Type is a Bollinger pass by value, and Doe, whose Type is a Bollinger pass by pointer.
If the CanFly
field is “optional” and the default value is “true”
The param
in the example code play as a request without CanFly
parameter
- Assign the variables of type “John” and “Doe”
- Use
json.Unmarshal
to convert the incoming json string to Struct - Convert to json strings via
json.Marshal
for easy reading and printing on the screen
The first line of the output is “John”, which using the boolean value as type.
When calling new(John)
will automatically bring in the zero value
.
And the default zero value of boolean is false.
And Name
and CanRnu
are overwritten with zero value because they are passed in.
But because CanFly
is optional and not passed in by the user, it is still false
We can’t identify the false is from user or from zero value.
If you use “pointer” as the type in Struct, you can see that the second line of CanFly
is null.
So you can set the default value to “true” later on.
This is what I learned about the default value problem when converting json strings to Struct, and how to solve it with a pointer.