[A Tour of Go Study Notes] 08 interface and error
Understanding Go interface and error using official tutorials
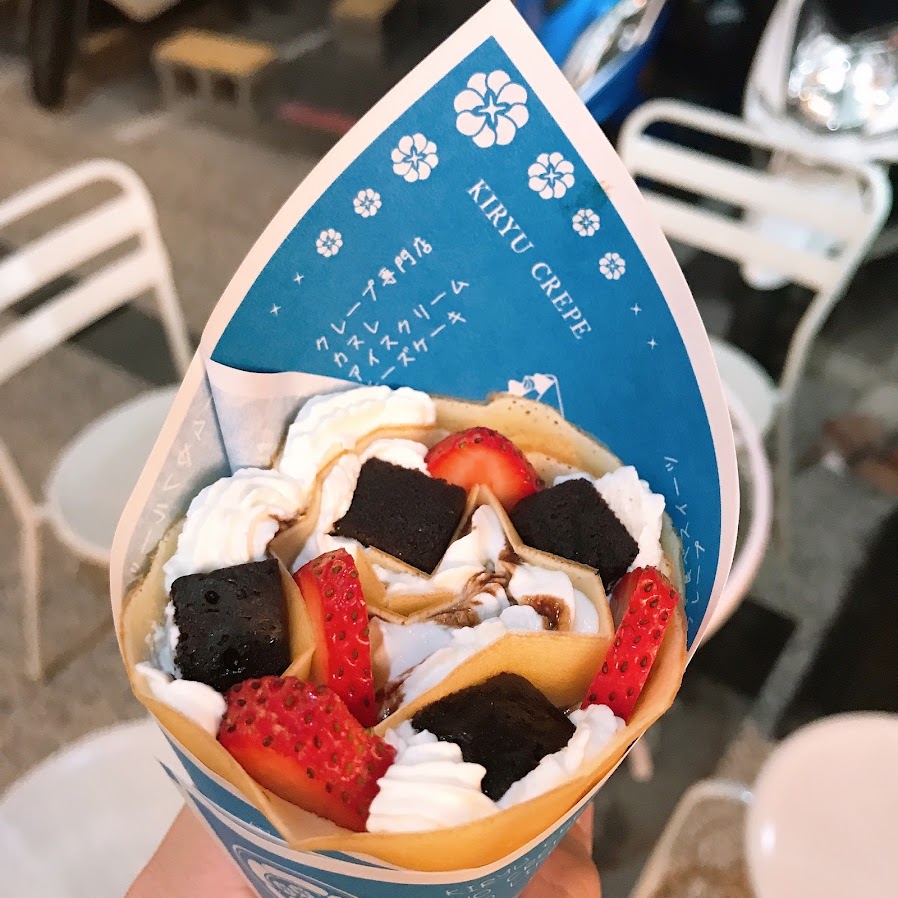
Entering the world of Golang, we first explore the basics of Golang using the official tutorial: A Tour of Go
.
This piece introduces interfaces and errors.
Interface
The concept is somewhat akin to PHP’s implementation
.
Defining the function name, accepted parameters with types, and the returned value along with its type.
Rather than defining implementation methods, it’s up to individual objects inheriting to implement them.
However, Go lacks an inheritance mechanism; there’s no need to explicitly use the implement
keyword to declare which interface it implements.
Regardless of the data type, as long as it implements the definitions within an interface, it automatically implements that interface.
An interface type defines a set of methods, and if an object implements all the methods of an interface, then that object implements the interface itself.
package main
import "fmt"
type I interface {
M()
}
type T struct {
S string
}
// This method means type T implements the interface I,
// but we don't need to explicitly declare that it does so.
func (t T) M() {
fmt.Println(t.S)
}
func main() {
var i I = T{"hello"}
i.M()
}
/*
>>> hello
*/
This feature is also known as Duck typing .
“When I see a bird that walks like a duck and swims like a duck and quacks like a duck, I call that bird a duck.”
It can be explained that when an object implements all the definitions of a duck interface, it’s considered a duck.
Error
In Go, error
is used to represent error states.
Similar to fmt.Stringer
, the error type is a built-in interface.
type error interface {
Error() string
}
Usually, functions return an error
, and checking if the value is nil
is used to handle errors.
i, err := strconv.Atoi("42")
if err != nil {
fmt.Printf("couldn't convert number: %v\n", err)
return
}
fmt.Println("Converted integer:", i)
When the error is nil
, it signifies success; a non-nil
error indicates a failure.