[A Tour of Go Study Notes] 04 Pointers and Structs
Understanding Go language pointers and structs using the official tutorial
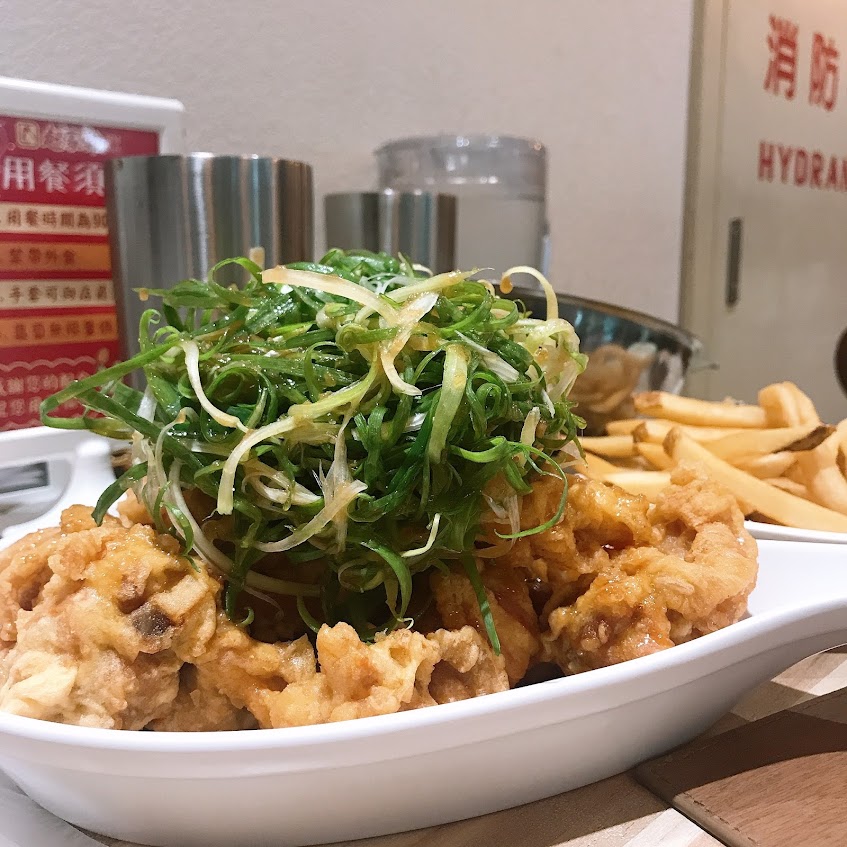
Entering the world of Golang, we first explore the basic usage of Golang using the official tutorial: A Tour of Go
.
This article introduces the usage of pointers and structs.
Pointers
Go has pointers, which represent the memory address of a value.
*T
represents a pointer to type T
, with a default value of nil
.
var p *int // p = nil
The &
symbol points to the “memory address” of the target.
i := 42
p := &i // p = 0xc000018038 = memory location of variable i
The *
operator retrieves the “value” represented by that pointer.
fmt.Println(*p) // Retrieves the value represented by pointer p, which is i
*p = 21 // Changes i indirectly by setting the value of pointer p
This is commonly referred to as “dereferencing” or “indirect referencing.”
package main
import "fmt"
func main() {
i, j := 42, 2701
p := &i // point to i
fmt.Println(*p) // read i through the pointer
*p = 21 // set i through the pointer
fmt.Println(i) // see the new value of i
p = &j // point to j
*p = *p / 37 // divide j through the pointer
fmt.Println(j) // see the new value of j
}
/*
>>> 42
>>> 21
>>> 73
*/
Structs
Similar to JavaScript objects, structs are collections of fields.
Variables store single values; when more complex concepts like coordinates are needed, structs can be used.
package main
import "fmt"
type Vertex struct {
X int
Y int
}
func main() {
fmt.Println(Vertex{1, 2})
}
/*
>>> {1 2}
*/
Even the way to access them is quite similar to JavaScript objects, using .
to access the contents within the struct.
Notice, here X
and Y
are both capitalized.
This signifies that the content is exported and can be accessed externally!
package main
import "fmt"
type Vertex struct {
X int
Y int
}
func main() {
v := Vertex{1, 2}
v.X = 4
fmt.Println(v.X)
}
/*
>>> 4
*/
The pointer operations mentioned earlier also apply to struct operations.
package main
import "fmt"
type Vertex struct {
X int
Y int
}
func main() {
v := Vertex{1, 2}
p := &v // p points to the memory location of v, indicating they refer to the same target
p.X = 1e9
fmt.Println(v)
}
/*
>>> {1000000000 2}
*/
Creating Structs
package main
import "fmt"
type Vertex struct {
X, Y int
}
var (
v1 = Vertex{1, 2} // Creates a struct of type Vertex
v2 = Vertex{X: 1} // Y:0 because int type defaults to 0
v3 = Vertex{} // X:0, Y:0
p = &Vertex{3, 2} // Creates a struct of type *Vertex
)
func main() {
fmt.Println(v1, v2, v3, p)
}
/*
>>> {1 2} {1 0} {0 0} &{3 2}
*/