[A Tour of Go Study Notes] 01 Packages and Functions
Explore the fundamentals of Go language's basic package structure and functions through the official tutorial.
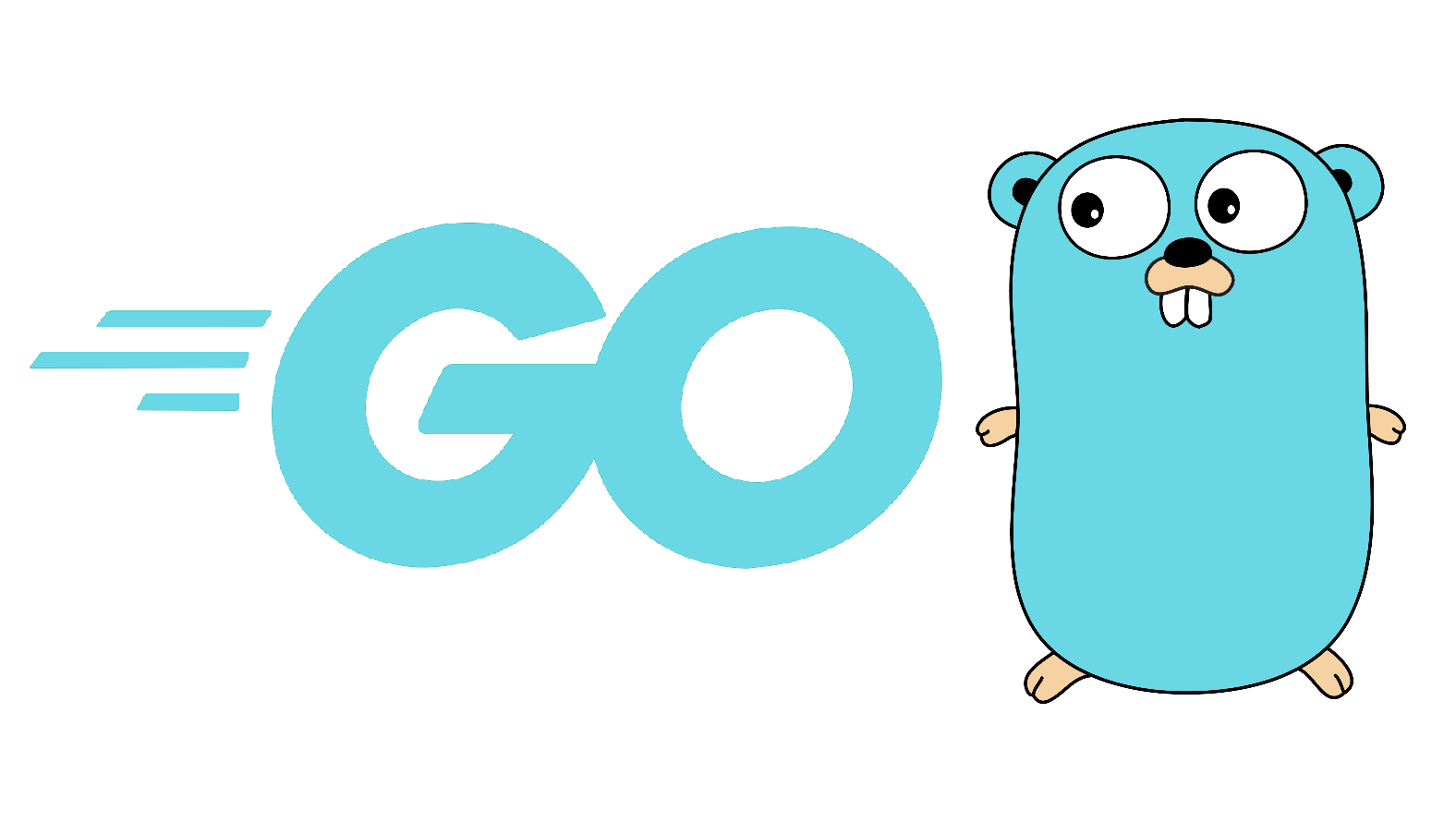
Entering the world of Golang for beginners, we start with the basics of Golang using the official tutorial: A Tour of Go
.
This piece covers the fundamental aspects of basic packages and functions in Golang.
Hello World
The unavoidable Hello World
starts off our journey.
package main
import "fmt"
func main() {
fmt.Println("Hello World")
}
Packages
Every Go program consists of packages.
The execution begins with the main
package.
Import
In the example below, two packages, fmt
and math/rand
, are imported.
According to convention, the package name matches the last element of the import path.
For instance, methods in math/rand
start with package rand
.
package main
import (
"fmt"
"math/rand"
)
func main() {
fmt.Println("My favorite number is", rand.Intn(10))
}
Apart from grouped imports within the import
parentheses, another way to import is by writing multiple lines for imports.
However, grouped imports are considered better practice.
package main
import "fmt"
import "math"
func main() {
fmt.Printf("Now you have %g problems.\n", math.Sqrt(7))
}
Exported Name
In Go, if a name starts with an uppercase letter, it indicates that it’s exported and can be used externally.
For example, Pizza
is an exported name, just like Pi
in the math
package.
However, pizza
and pi
are not exported names because they don’t start with an uppercase letter.
When using a package, you can only access the content of exported names.
Non-exported content cannot be accessed externally!
package main
import (
"fmt"
"math"
)
func main() {
fmt.Println(math.Pi)
}
Functions
Similar to other languages, a function can accept zero or more parameters.
The add
function in the example takes two int
parameters. Note that the type is defined after the parameter.
package main
import "fmt"
func add(x int, y int) int {
return x + y
}
func main() {
fmt.Println(add(42, 13))
}
/*
>>> 55
*/
If consecutive parameters have the same type, you can omit the type declaration for preceding parameters and specify it only for the last one, changing x int, y int
to x, y int
.
package main
import "fmt"
func add(x, y int) int {
return x + y
}
func main() {
fmt.Println(add(42, 13))
}
/*
>>> 55
*/
Returning Multiple Values
A function can return any number of results.
package main
import "fmt"
func swap(x, y string) (string, string) {
return y, x
}
func main() {
a, b := swap("hello", "world")
fmt.Println(a, b)
}
/*
>>> world hello
*/
You can also name the return values.
Naming the return values at the top of the function treats them as variables within the function.
A return
statement without any arguments returns the named return values directly, known as a “naked” return.
Named return values should have meaningful names and can be used as documentation.
Naked returns should be used in concise functions as using them in longer functions may impact readability.
package main
import "fmt"
func split(sum int) (x, y int) {
x = sum * 4 / 9
y = sum - x
return
}
func main() {
fmt.Println(split(19))
}
/*
>>> 4 5
*/