TypeScript Generics
Using TypeScript generics to write reusable and type-safe code
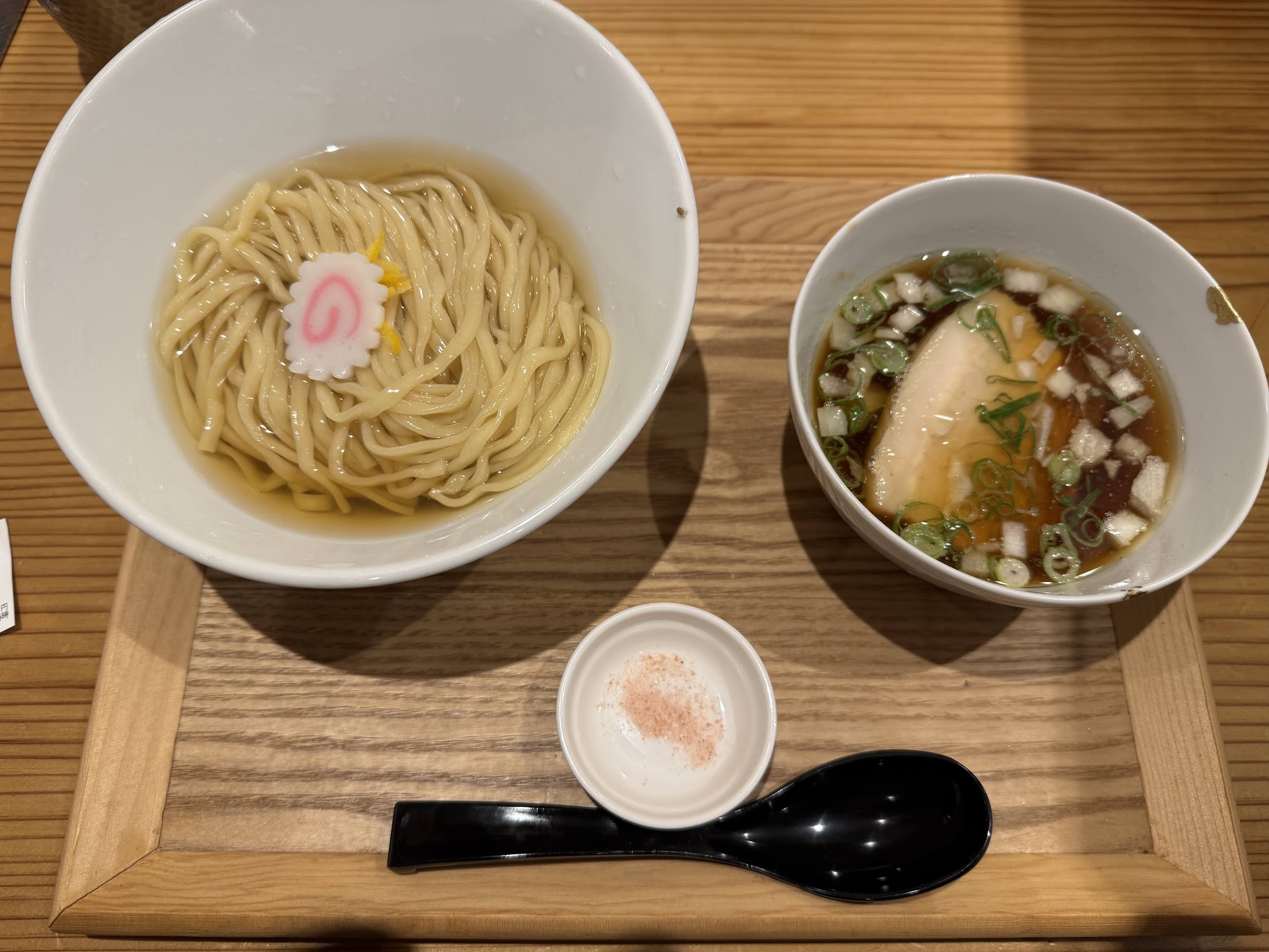
Introduces TypeScript generics, demonstrating how to enhance code reusability by defining flexible methods, interfaces, and classes that adapt to different types.
The ability to define methods, interfaces, or classes without specifying data types in advance, and determining the types when used.
// Original method: requires writing similar code for different types
const pushStrArr = (arr: string[], item: string): string[] => {
arr.push(item);
return arr;
};
const pushNumArr = (arr: number[], item: number): number[] => {
arr.push(item);
return arr;
};
// Using generics, where "T" is just a placeholder and must be uppercase
const pushArr = <T>(arr: T[], item: T): T[] => {
arr.push(item);
return arr;
};
// Declare the type to be used by specifying <T>
pushArr<number>([1, 2, 3, 4], 5);
pushArr<string>(["a", "b", "c"], "d");
// Using generics with multiple types
function swapGeneric<T, U>(tuple: [T, U]): [U, T] {
return [tuple[1], tuple[0]]; // Swap positions
}
/**
Declare T = string, U = number
Subsequent usage of T and U will be recognized as these types
**/
const res = swapGeneric<string, number>(["123", 456]);
// res = [456, '123']