Class and Interface in TypeScript
Dive into TypeScript Classes and Interfaces with syntax examples.
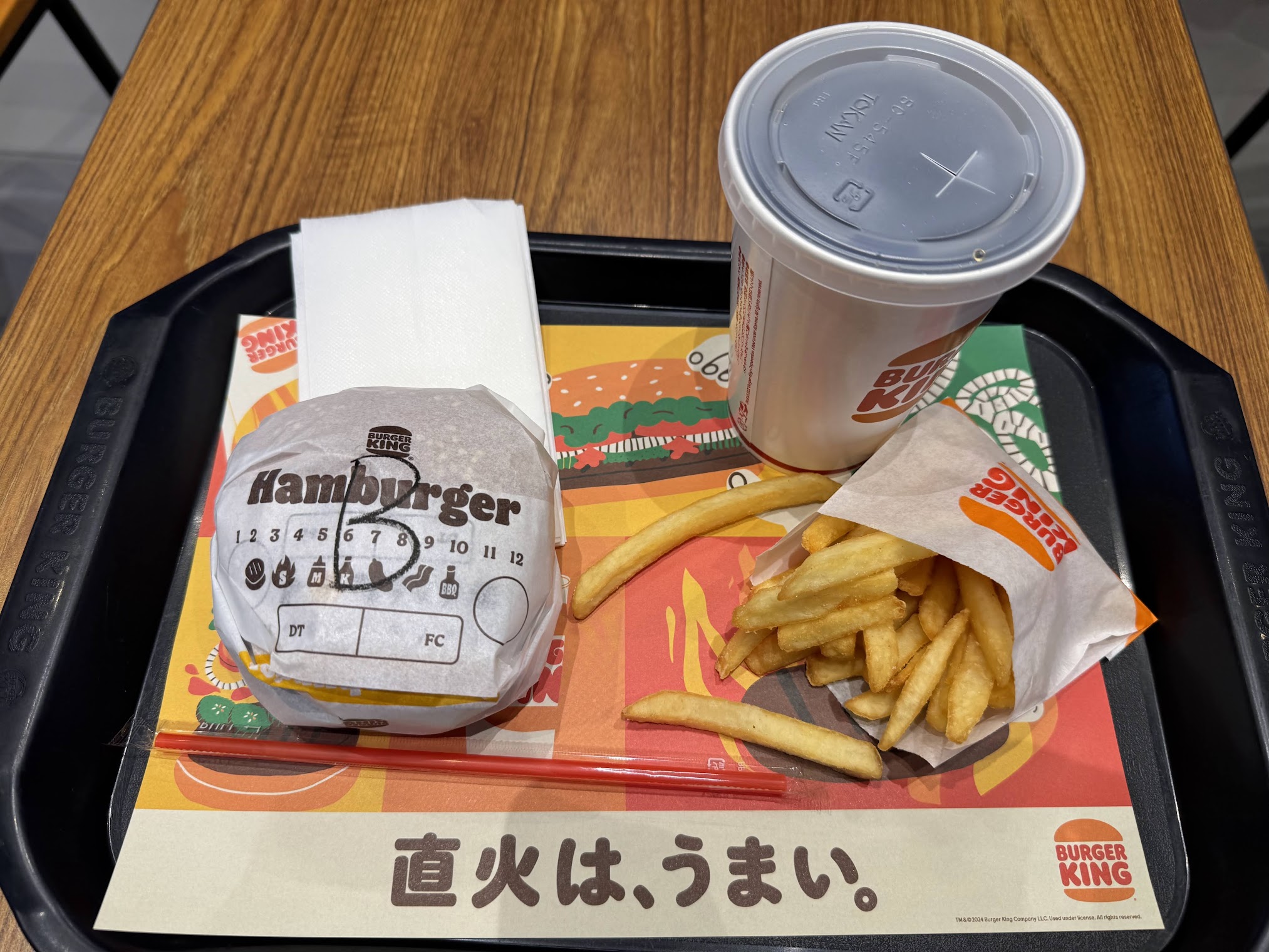
Introduces the basic concepts of TypeScript Classes and Interfaces.
It also covers class implementation and interface inheritance examples.
Interface
Defines arbitrary structures or types.
Basic Definition
interface publicPoint {
w?: number; // Optional properties do not need to be at the end
x: number | string;
y: number;
}
// Interfaces can be merged but cannot overwrite existing properties
interface publicPoint {
z: number;
}
// Property inheritance
interface Point1 extends publicPoint {
a?: number;
}
interface Point2 extends publicPoint {
b: string;
c: string;
}
interface privatePoint {
d: number;
}
// Inherit multiple interfaces simultaneously
interface Point3 extends publicPoint, privatePoint {
e: number;
}
const myPoint1: Point1 = { x: 0, y: 0, z: 0 };
const myPoint2: Point2 = { x: 0, y: 0, z: 0, b: "oh", c: "nyo" };
const myPoint4: Point3 = { x: 0, y: 0, z: 0, d: 0, e: 0 };
Function Definition
interface Func {
(num1: number, num2: number): number;
}
const addFunc: Func = (arg1, arg2) => arg1 + arg2;
Index Signature
When defined as an index type, the original array will no longer have methods from the array prototype. For example: calling role1.length
will throw an error.
interface Role {
[id: number]: string;
}
const role1: Role = ["admin", "user"];
const role2: Role = {
0: "admin",
1: "user",
5: "author",
};
Avoiding Excess Property Checks
When using an interface, passing extra parameters may cause an error due to excess properties.
There are three methods to avoid Excess Property Checks.
1. Type Assertion
interface MyType {
color: string;
}
const getColor = (myType: Mytype) => {
return `${myType.color}`;
};
getColor({
color: "yellow",
type: "color",
num: 0,
} as MyType); // Declare it explicitly as MyType
2. Index Signature
Recommended approach
interface MyType {
color: string;
[prop: string]: any; // Accept additional properties
}
const getColor = (myType: Mytype) => {
return `${myType.color}`;
};
getColor({
color: "yellow",
type: "color",
num: 0,
group: [],
skill: () => {},
});
3. Type Compatibility
Not recommended
interface MyType {
color: string;
}
// Destructure to extract only required properties
const getColor = ({ color }: Mytype) => {
return `${color}`;
};
const option = { color: "yellow", size: 12 };
getColor(option);
Class
class Person {
name = "Rui"; // Default is public
private age = 30; // Private property
getAge() {
return this.age;
}
}
const person = new Person();
// Inheritance
class Person1 extends Person {
// When an object is created using `new`, the constructor is executed first, even if not explicitly defined.
constructor() {
super(); // Calls the parent class constructor. If a constructor is defined, `super` must be called.
console.log(super.getAge()); // Calls the parent class method
console.log(this.getAge()); // Calls the overridden subclass method
}
// The subclass overrides the parent method
getAge() {
return 18;
}
}
const person1 = new Person1();
Class Implementing an Interface
interface FoodInterface {
type: string;
}
class ChineseFoodClass implements FoodInterface {
constructor(public type: string) {}
}
// Equivalent to
class JapaneseFoodClass implements FoodInterface {
type: string;
constructor(arg: string) {
this.type = arg;
}
}
Interface Extending a Class
Interfaces inherit types but not implementations.
Interfaces inherit private and protected properties, but they can only be implemented by the class itself or its subclasses.
class Person {
protected name = "Rui";
private nickName = "Ray";
getName() {
return this.name + this.nickName;
}
}
interface I extends Person {}
// Use `extends` between classes and between interfaces
// Use `implements` between a class and an interface
class C extends Person implements I {
getName() {
return this.name + " new Class C";
}
}
const newInstance = new C();
console.log(newInstance.getName());
// Rui new Class C