Basic Data Type in TypeScript
Summarizes the basic types and syntax applications in TypeScript
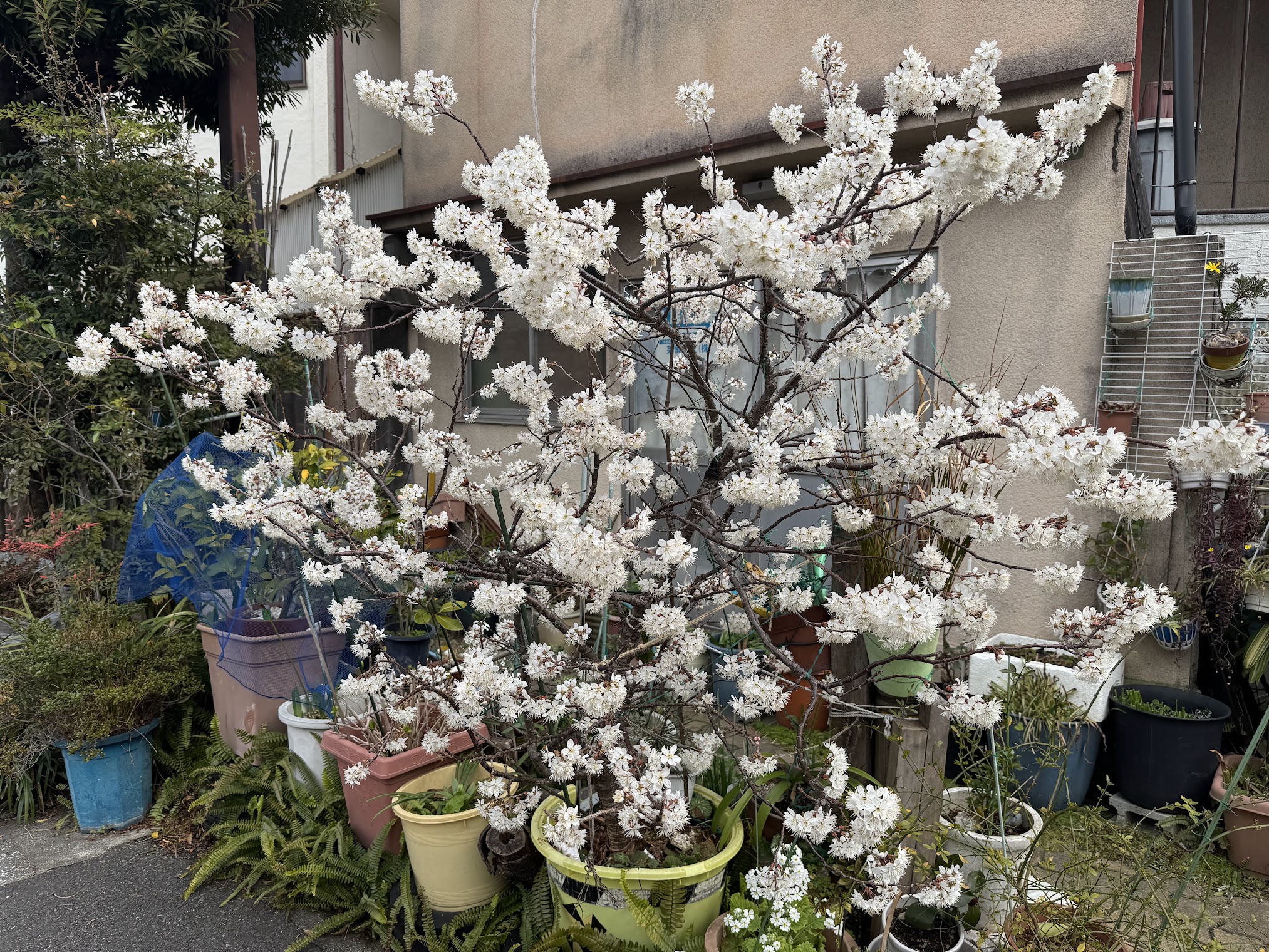
Introduction to TypeScript’s basic types and syntax examples,
including examples of basic function syntax, arrow functions, anonymous functions, and function overloading.
String
Constant const
Cannot be reassigned!
Variable let
Can override the original value
// string
const msg: string = "hello ts!";
let msg1: string = "123";
const msgStr = `$(msg} $(msg1}`;
console.log(msgStr); // hello ts! 123
Boolean
// boolean
const bool: boolean = true;
any
Equivalent to var
in ES5, representing any type.
Can directly call any method on the Prototype Chain.
let x: any;
x = 123;
x = "123";
x = () => {};
x.toFix(2);
x.join("-");
void
No type, can only be assigned to undefined
.
let x: void;
x = undefined;
unknown
Unknown type
You must perform type checking before calling any method on the Prototype Chain.
let x: unknown;
x = "123123";
x = 123;
x = undefined;
x = null;
if (typeof x === "number") {
x.toFixed(2);
} else if (typeof x === "object" && x instanceof Array) {
x.join("-");
}
tuple
An array with fixed types and length
const idolInfo: [string, string, number] = ["JP", "Gen2", 17];
enum
Lists all possible constants, can be used like an array.
enum Direction {
Up,
Down,
Left,
Right,
}
console.log(Direction.Up);
console.log(Direction[0]);
console.log(Direction[1]);
console.log(Direction[5]);
/**
0
Down
Left
undefined
**/
Indexes are continuous and can be modified.
enum Direction {
Up = 100,
Down,
Left,
Right,
}
console.log(Direction.Up);
console.log(Direction.Down);
console.log(Direction.Left);
console.log(Direction[103]);
/**
100
101
102
Right
**/
object
// object
const obj: object = {};
const obj1: {} = {};
const obj2: { msg: string; num: number } = { msg: "hello", num: 0 };
// Optional properties use "?" and must be placed at the end.
const obj3: { msg: string; num?: number } = { msg: "hello" };
// Modifying object properties later
obj3.num = 0;
obj3.num = 123;
array
const arr: [] = [];
const arr1: Array<string> = ["111"];
const arr3: string[] = ["333"];
// Union Types
const arr2: Array<string | number> = ["222", 222];
const arr4: (string | number)[] = ["444", 444];
function
Basic syntax
function add(arg1: number, arg2: number): number {
return arg1 + arg2;
}
// Type inference
const result = add(1, 2);
Arrow function
const add = (arg1: number, arg2: number): number => {
return arg1 + arg2;
};
// Type inference
const result = add(1, 2);
Anonymous function
JavaScript syntax:
var fn = function {}
const fn = () => {}
const add = (arg1, arg2) => arg1 + arg2
Add types to become TypeScript syntax:
const add: (arg1: number, arg2: number) => number = (
arg1: number,
arg2: number
) => arg1 + arg2;
Function overloading
Only function
can be used for definition, arrow functions cannot be used!
Function overloading allows creating multiple methods with the same name but different parameter types or counts.
By providing multiple function type definitions, the compiler will handle the function calls accordingly.
// Declare acceptable variable types and return types
function handleDate(x: string): string[];
function handleDate(x: number): string;
// Implementation logic
function handleDate(x: any): any {
if (typeof x === "string") {
return x.split("");
} else {
return x.toString().split("").join("-");
}
}
handleDate("abc").join("-"); // Returns string[], so array methods can be used
handleDate(123).length; // Returns string, so only string methods can be used
handleDate(false); // Error, because this overload is not declared