TypeScript 的基本資料型別
TypeScript 的基本資料型別與語法範例
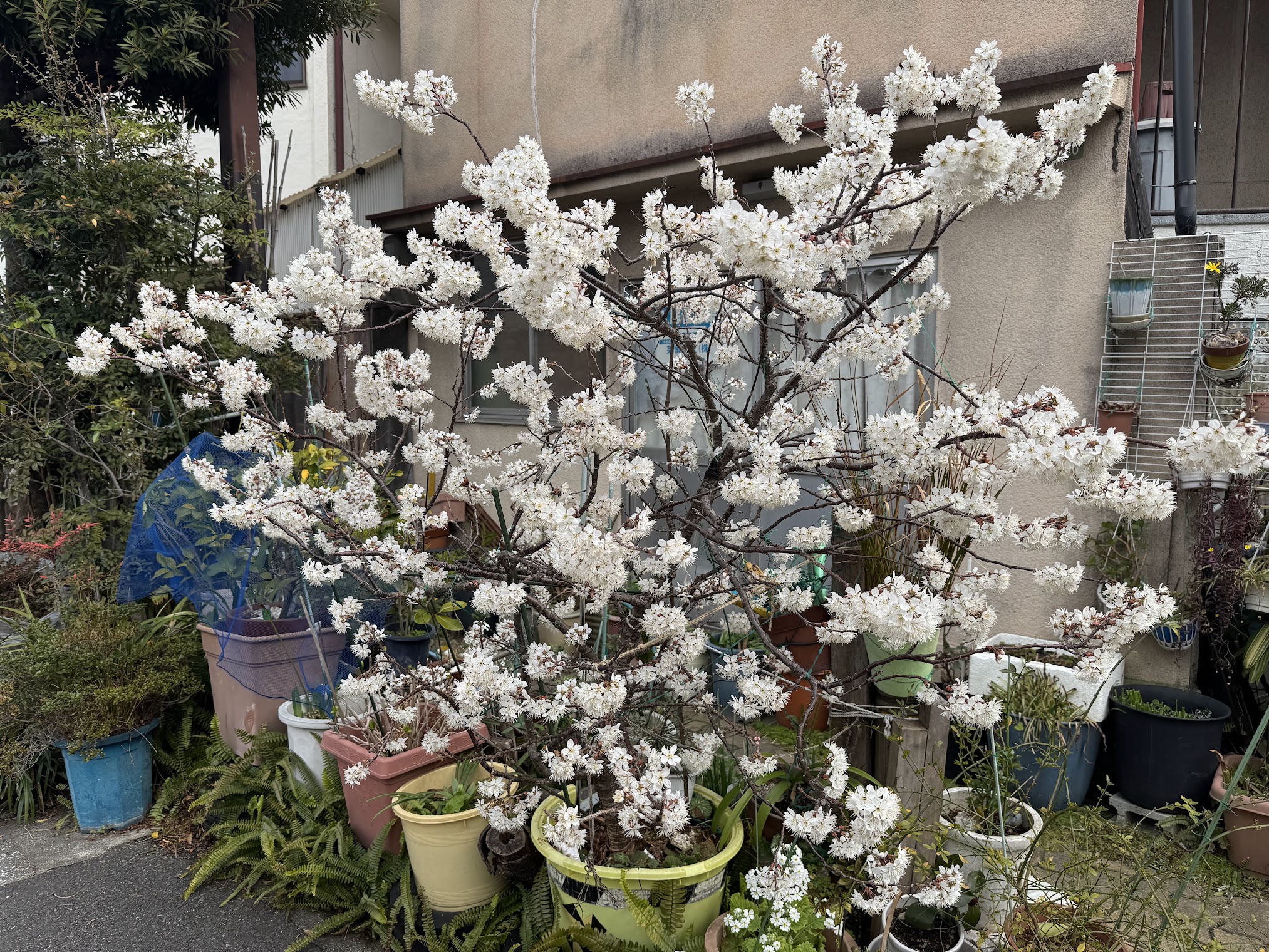
介紹 TypeScript 的基本型別與語法範例
包含基本函式語法、箭頭函式、匿名函式及函式多載的範例
字串
常數 const
不可重新賦值!
變數 let
可以覆蓋原有值
// string
const msg: string = "hello ts!";
let msgl: string = "123";
const msgStr = `$(msg} $(msg1}`;
console.log(msgStr); // hello ts! 123
布林
// boolean
const bool: boolean = true;
any
等同於 ES5 的 var
代表任意型別
可以直接呼叫所有原型鏈(Prototype Chain)上的方法
let x: any;
x = 123;
x = "123";
x = () => {};
x.toFix(2);
x.join("-");
void
沒有類型,只能賦值成 undefined
let x: void;
x = undefined;
unknown
未知類型
必須通過類型判斷後才能呼叫原型鏈(Prototype Chain)上的方法
let x: unknown;
x = "123123";
x = 123;
x = undefined;
x = null;
if (typeof x === "number") {
x.toFixed(2);
} else if (typeof x === "object" && x instanceof Array) {
x.join("-");
}
tuple(元組)
固定類型和長度的陣列
const idolInfo: [string, string, number] = ["JP", "Gen2", 17];
enum(枚舉)
列出所有可能的常數,可以當作陣列使用
enum Direction {
Up,
Down,
Left,
Right,
}
console.log(Direction.Up);
console.log(Direction[0]);
console.log(Direction[1]);
console.log(Direction[5]);
/**
0
Down
Left
undefined
**/
索引是連續且可以修改的
enum Direction {
Up = 100,
Down,
Left,
Right,
}
console.log(Direction.Up);
console.log(Direction.Down);
console.log(Direction.Left);
console.log(Direction[103]);
/**
100
101
102
Right
**/
object
// object
const obj: objeft = (}
const obj1: (} = {}
const obj2: { msg: string, num: number } = { msg: 'hello', num: 0 }
// 可選屬性使用「?」並且擺在最後一個
const obj3: { msg: string, num?: number } = { msg: 'hello' }
// 在後續修改物件中的值
obj3.num = 0
obj3.num = 123
array
const arr: [] = [];
const arr1: Array<string> = ["111"];
const arr3: string[] = ["333"];
// Union Types(聯合型別)
const arr2: Array<string | number> = ["222", 222];
const arr4: (string | number)[] = ["444", 444];
function
基本語法
function add(arg1: number, arg2: number): number {
return arg1 + arg2;
}
// 型別自動推斷
const result = add(1, 2);
箭頭函數
const add = (arg1: number, arg2: number): number => {
return arg1 + arg2;
};
// 型別自動推斷
const result = add(1, 2);
匿名函數
Javascript 語法:
var fn = function {}
const fn = () => {}
const add = (arg1, arg2) => arg1 + arg2
加入型別成為 typescript 語法
const add: (arg1: number, arg2: number) => number = (
arg1: number,
arg2: number
) => arg1 + arg2;
函式多載(function overloading)
只能使用 function 定義,不能使用箭頭函數!
函式重載是使用相同名稱和不同參數數量或類型創建多個方法的一種能力
藉由為同一個函式提供多個函式類型定義來進行函式重載 編譯器會根據這個列表去處理函式的調用
// 聲明可以接收的變數和返回型別
function handleDate(x: string): string[];
function handleDate(x: number): string;
// 實作處理邏輯
function handleDate(x: any): any {
if (typeof x === "string") {
return x.split("");
} else {
return x.toString().split("").join("-");
}
}
handleDate("abc").join("-"); // 回傳是 string[] 所以可以使用 array 的方法
handleDate(123).length; // 回傳是 string,只能使用 string 的方法
handleDate(false); // 錯誤,因為沒有聲明多載